Arrow functions and this keyword
An arrow function is an
Before actually going to the depth of arrow functions lets take a quick look on how regular function expressions work
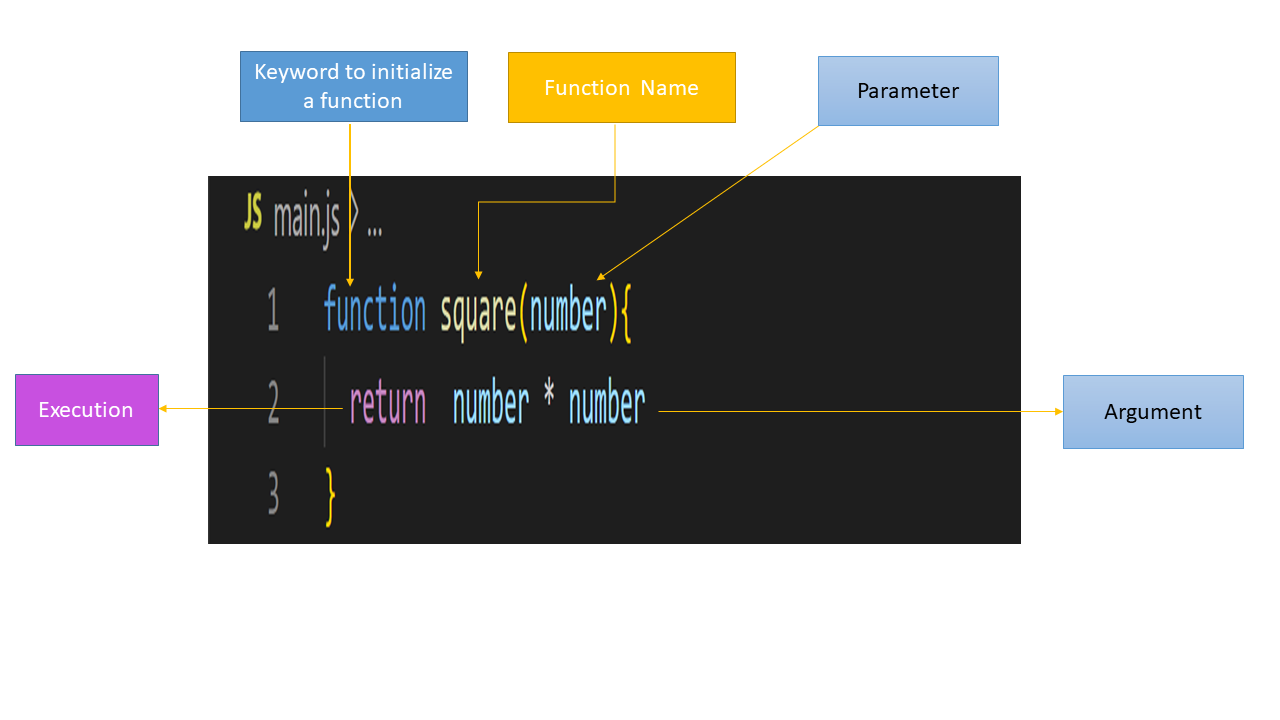
Functions defined in short could be, a reusable piece of code that is designed to perform a particular task, as shown in image 2.1 the function square is taking a numeric parameter and returning its square value thus, performing a task which can be reused whenever called.
Let’s take a look on an Arrow function which takes same parameters and arguments mentioned in image 2.1
Arrow Function Syntax
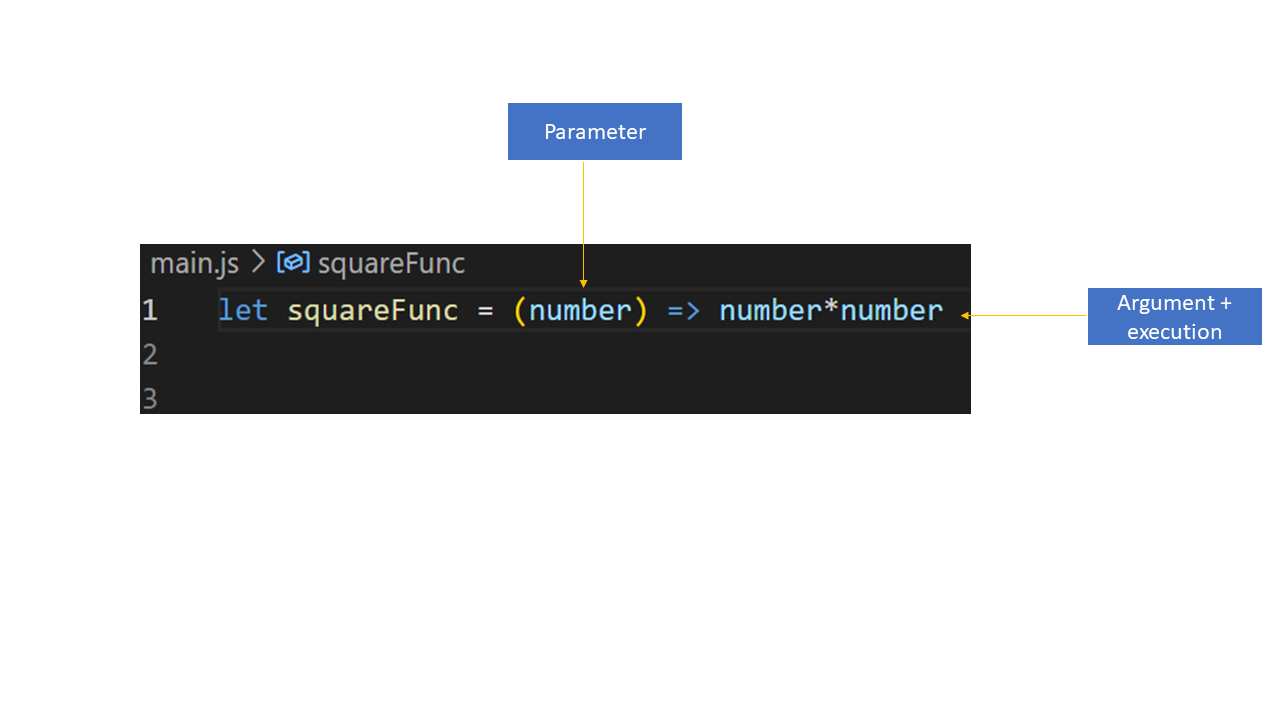
Here, both regular function (img 2.1 ) and es6 function(img 2.2 ) are going to return the same output after being called
- If the arrow function is inline then the “return ” statement is not required to execute the argument
- Usage of curly braces(“{}”) , parenthesis(“()”) or return statement becomes optional according to the need of developer
- An arrow function is usually assigned to a variable
Some syntactical differences are mentioned below
But what makes arrow functions different from regular functions ?
If you are thinking that precise syntax is the distinctive point here then you are probably wrong
Because what actually makes the fundamental difference is the way through which arrow functions handlethis keyword
A regular function expression binds its own “this” keyword which usually refers to the global or window object unless it is invoked as an method in simple words the assignment value of this inside a function depends on how it is invoked like it will refer to its parent object if invoked as a method but there comes an unexpected case scenario when we try to use “this” inside nested function or in an inner function of a method than it refers to the window object instead of pointing to its parent
const myObj = {
name:"Sam",
hobbies: ["code", "design", "read book"],
message: function (){
this.hobbies.forEach(function innerFunc(hobbies)
{
console.log( `${this.name} likes to ${hobbies}`)
})
}
}
myObj.message() //output "undefined have hobbies…… *3"
So,referencing to the above-stated code snippet if you try to get the value of this from the inner function of a regular function/method then you will get “window object” as result( “global” in node.js) instead of getting the expected parent object(myObj in the given snippet) meaning this keyword treats inner/ nested functions inside a method as regular functions only
And to sort out the confusing referencing issue of ‘’this’’, Arrow Functions was introduced in ES6 we are going to take same example , same naming conventions we will only change out our innerFunc() function from regular to arrow function and will get the desired output i.e. the reference to our parent object (myObj)
const myObj = {
name:"Sam",
hobbies: ["code", "design", "read book"],
message: function (){
this.hobbies.forEach( innerFunc = (hobbies) => {
console.log (`${this.name} likes to ${hobbies}`)
}
)
}
}
myObj.message() // output : sam likes to code.....design etc
Conclusion
It wouldn't be wrong to say that arrow functions were mainly introduced to eliminate the chaos created by this keyword though JS already had various solutions like storing value of this inside a variable outside the inner function or using .bind() but arrow function mainly reduces the amount of code and user readability that’s why it is always preferable to use arrow functions especially when using methods like .map() , .forEach() etc .
©Shubhendu Sen